What are Python dictionaries?
A dictionary is one of Python’s primary data structures. In essence, a dictionary (also shortened as dict) is a collection of key-value pairs, similar to an actual dictionary (like the one pictured above). When you look up a word in a dictionary, you find a corresponding definition. Similarly, in a Python dictionary, each key has a corresponding value.
In dictionaries, one key can have only one value. However, the value can be anything from a single number or character, to lists, tuples, other dictionaries, or even functions!
Creating a Dictionary
Dictionaries can be created several ways. The most straightforward way is below using curly braces.
# method 1)
mapper = {“a”: 1, “b”: 2, “c”:3}
In this example, we create a dictionary with three elements – that is, three key-value pairs. The keys are “a”, “b”, and “c”, while the corresponding values are 1, 2, and 3.
Getting the keys and values of a dictionary
We can get the keys of our dictionary using the keys method.
mapper.keys()
Similarly, we can get the values of a dictionary using the values method.
mapper.values()
Adding new key-value pairs
We can add new key-value pairs to a dictionary using bracket notation.
mapper[“d”] = 4
mapper[“e”] = 5
Likewise, if we want to refine a key-value pair, we also use bracket notation.
mapper[“a”] = 10

Removing key-value pairs
Key-value pairs can be removed in a couple of different ways. The first is using the pop method.
mapper.pop(“e”)
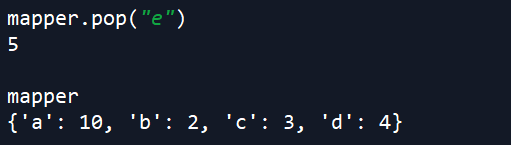
Using the pop method both removes the key-value pair and returns the value corresponding to the key input into the method. Alternatively, we can remove a key-value pair using Python’s del method. This way does not return any value.
del mapper[“d”]
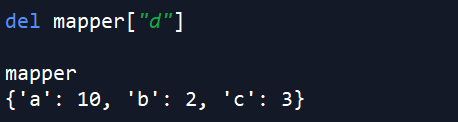
How to combine two Python dictionaries
Dictionaries can be combined using the update method. For example, we currently have:
mapper = {“a”, “b”, “c”}
Now let’s create another dictionary called extra:
extra = {“this”: “is”, “another”:”dictionary”}
Next, let’s combine mapper and extra.
mapper.update(extra)
Running the update method here will update mapper to also have the key-value pairs in extra.
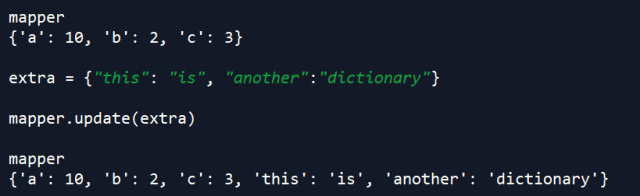
The items method
We can convert a dictionary into a list of tuples using the items method. Here, each tuple will be a key-value pair. The first line below will create a dict_items object, which is iterable, so we can loop over the key-value pairs in this object. However, this object is not indexable – i.e. trying to run mapper.items()[0] will result in an error. However, if we wanted to reference key-value pairs by index, we can convert this to a list. In practice, we usually would not need to do this conversion.
# get key-value tuples in dictionary
mapper.items()
# convert to list
list(mapper.items())
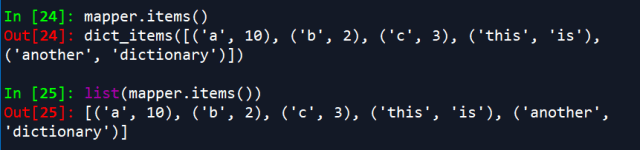
The items method is especially useful when iterating over a dictionary, or when creating a dictionary comprehension, which we’ll discuss next.
Suppose we have dictionary of filenames – each key is a filename, and each value is a new name that we want to give for the key. Here, we can loop over the items (key-value pairs) in the dictionary and change each file name.
import os
files = {“file1.txt”: “new_file.txt”, “file2.txt”, “new_file2.txt”, “file3.txt”, “new_file3.txt”}
for key,val in files.items():
os.rename(key, val)
Visit TheAutomatic.net to download ready-to-use code, and read the rest of the article: http://theautomatic.net/2020/04/15/all-about-python-dictionaries/
Disclosure: Interactive Brokers
Information posted on IBKR Campus that is provided by third-parties does NOT constitute a recommendation that you should contract for the services of that third party. Third-party participants who contribute to IBKR Campus are independent of Interactive Brokers and Interactive Brokers does not make any representations or warranties concerning the services offered, their past or future performance, or the accuracy of the information provided by the third party. Past performance is no guarantee of future results.
This material is from TheAutomatic.net and is being posted with its permission. The views expressed in this material are solely those of the author and/or TheAutomatic.net and Interactive Brokers is not endorsing or recommending any investment or trading discussed in the material. This material is not and should not be construed as an offer to buy or sell any security. It should not be construed as research or investment advice or a recommendation to buy, sell or hold any security or commodity. This material does not and is not intended to take into account the particular financial conditions, investment objectives or requirements of individual customers. Before acting on this material, you should consider whether it is suitable for your particular circumstances and, as necessary, seek professional advice.