Excerpt
What is Blockchain?
A blockchain essentially is a database that is distributed across multiple nodes/computers. It is made up of ‘blocks’ of information, and each block is ‘chained’ to its previous block. Blocks? Chains? Let’s break it down further.
Blocks, chains and hashes
A block is made up of several records, like a table in a regular database or a spreadsheet. But what makes it different from a traditional database is that each block is connected or chained to the previous block. We will see how this ‘chaining’ happens in a while.
Now, before we move any further, let us briefly touch upon what a hash is. Just like fingerprints are used to identify human beings, a hash is a string of seemingly random numerical values that represents any file – an image, text, audio, etc. Every time you create a hash of a file – you will get the same value.
However, even a minor modification to the file, be it whitespace, or even a comma, will change the hash.
There are different types of hashing algorithms, but the one usually used in blockchain is SHA256. Here, SHA means Secure Hash Algorithm.
In Python, we can use the ‘hashlib‘ module to generate hashes for a file/string. This module supports many different types of hashing algorithms.
import hashlib
for hash in hashlib.algorithms_guaranteed:
print(f'{hash} algorithm is available.')
Using hashlib.py hosted with ❤ by GitHub
sha1 algorithm is available.
sha3_256 algorithm is available.
shake_256 algorithm is available.
blake2b algorithm is available.
sha3_512 algorithm is available.
sha256 algorithm is available.
sha384 algorithm is available.
md5 algorithm is available.
sha224 algorithm is available.
sha3_224 algorithm is available.
shake_128 algorithm is available.
sha512 algorithm is available.
blake2s algorithm is available.
sha3_384 algorithm is available.
Here is an illustration of generating a SHA256 hash for a string in Python, and how the hash changes with tiny changes in the string.
string = ["Hello World!", "Hello World", "hello World!", "hello world!"]
for s in string:
hash = hashlib.sha256(s.encode()).hexdigest()
print(f'The hash for {s} is {hash}.')
Generating a SHA256 hash for a string.py hosted with ❤ by GitHub
The hash for Hello World! is 7f83b1657ff1fc53b92dc18148a1d65dfc2d4b1fa3d677284addd200126d9069.
The hash for Hello World is a591a6d40bf420404a011733cfb7b190d62c65bf0bcda32b57b277d9ad9f146e.
The hash for hello World! is e4ad0102dc2523443333d808b91a989b71c2439d7362aca6538d49f76baaa5ca.
The hash for hello world! is 7509e5bda0c762d2bac7f90d758b5b2263fa01ccbc542ab5e3df163be08e6ca9.
As we can see above, even a minor change in the string leads to a different hash.
Each block contains the data for that block along with the hash of the previous block. For the first block of the chain, called the ‘Genesis’ block, the value of the previous hash is a string of all zeros.
The second block contains the hash of the genesis block and the data, and the third block contains the hash of the second block and data, etc.
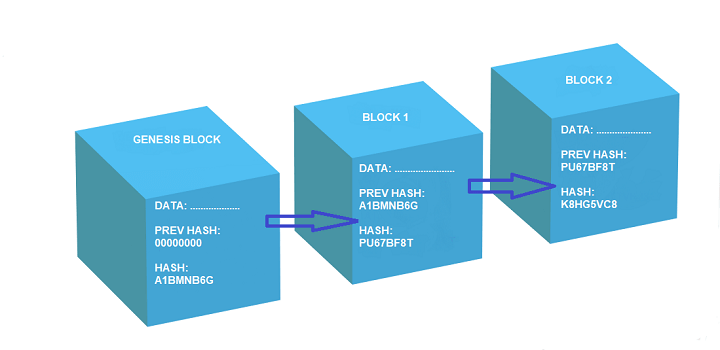
Representative image for a blockchain
Here is an example of a simple blockchain in Python:
import hashlib
import datetime as dt
# Define a block class
class block:
def __init__(self, time, data, prev_hash):
self.timestamp = time
self.data = data
self.prev_hash = prev_hash
self.hashvalue = self.generate_hash()
def generate_hash(self):
data = str(self.timestamp) + str(self.data) + str(self.prev_hash)
hashvalue = hashlib.sha256(data.encode()).hexdigest()
return hashvalue
# Create the genesis block with previous hash value as 0
def create_genesis_block():
timestamp = dt.datetime.now()
data = 'Genesis block'
prev_hash = '0'
return block(time=timestamp, data=data, prev_hash=prev_hash)
# Generate all later blocks in the blockchain
def create_block(prev_block, sender, receiver, amount):
timestamp = dt.datetime.now()
data = f'Sender: {sender}\nReceiver: {receiver}\nAmount: {amount}'
print('Data for this block is:\n',data)
prev_hash = prev_block.hashvalue
return block(time=timestamp, data=data, prev_hash=prev_hash)
# Let's create a simple blockchain with the genesis block
our_first_blockchain = [create_genesis_block()]
# The genesis block will be the previous block for the next block of the blockchain
prev_block = our_first_blockchain[0]
# Let us create a blockchain with 10 blocks
num_blocks = 10
for i in range(1, num_blocks+1):
next_block = create_block(prev_block, sender='Sender'+str(i), receiver='Receiver'+str(i), amount=i*1000)
our_first_blockchain.append(next_block)
print(f'Block number {i} is added to the blockchain. \nIts hash is {next_block.hashvalue}.\nHash of previous block is {next_block.prev_hash}.\n\n')
prev_block = next_block
Example of creating a Blockchain using Python.py hosted with ❤ by GitHub
Data for this block is:
Sender: Sender1
Receiver: Receiver1
Amount: 1000
Block number 1 is added to the blockchain.
Its hash is dc19a1fa6ece23fec46ba93c14ea351bf0eea7246e7051bd493727109bd3fc22.
Hash of previous block is 41bc2398bddbf8813f63c1f33e936982770a97388f01f58e0558ca9ad8abbd93.
Data for this block is:
Sender: Sender2
Receiver: Receiver2
Amount: 2000
Block number 2 is added to the blockchain.
Its hash is faa7596e965277014878f3c1016f4c55af75524f06972c6f0c0830fd70fcb798.
Hash of previous block is dc19a1fa6ece23fec46ba93c14ea351bf0eea7246e7051bd493727109bd3fc22.
Data for this block is:
Sender: Sender3
Receiver: Receiver3
Amount: 3000
Block number 3 is added to the blockchain.
Its hash is 9353c830b5fdfc1534ddd4c459d5aa7cef9f187c578fed68ca149f590cefcb82.
Hash of previous block is faa7596e965277014878f3c1016f4c55af75524f06972c6f0c0830fd70fcb798.
Data for this block is:
Sender: Sender4
Receiver: Receiver4
Amount: 4000
Block number 4 is added to the blockchain.
Its hash is 17d753bb661639ef1ff95664d51a19d183a718c55824436eefe380ba693b5e0f.
Hash of previous block is 9353c830b5fdfc1534ddd4c459d5aa7cef9f187c578fed68ca149f590cefcb82.
Data for this block is:
Sender: Sender5
Receiver: Receiver5
Amount: 5000
Block number 5 is added to the blockchain.
Its hash is 31421253ef41c82bb65fb46cc95933016c608cd4c46b27d8a670f89637694673.
Hash of previous block is 17d753bb661639ef1ff95664d51a19d183a718c55824436eefe380ba693b5e0f.
Data for this block is:
Sender: Sender6
Receiver: Receiver6
Amount: 6000
Block number 6 is added to the blockchain.
Its hash is affaaf4e8589071fda3b9229a96fcd4515934a48e72822106998492c9e092694.
Hash of previous block is 31421253ef41c82bb65fb46cc95933016c608cd4c46b27d8a670f89637694673.
Data for this block is:
Sender: Sender7
Receiver: Receiver7
Amount: 7000
Block number 7 is added to the blockchain.
Its hash is c982a9bdd32d4f39ae1a9cf7a4db3433dbbb69aa79fd3c6324c0a1bc6288d6c2.
Hash of previous block is affaaf4e8589071fda3b9229a96fcd4515934a48e72822106998492c9e092694.
Data for this block is:
Sender: Sender8
Receiver: Receiver8
Amount: 8000
Block number 8 is added to the blockchain.
Its hash is 3294043edb16abe51417fd1ba2d3ecdc497d11c897fdcbc96d6edc8ada661c26.
Hash of previous block is c982a9bdd32d4f39ae1a9cf7a4db3433dbbb69aa79fd3c6324c0a1bc6288d6c2.
Data for this block is:
Sender: Sender9
Receiver: Receiver9
Amount: 9000
Block number 9 is added to the blockchain.
Its hash is eb21c2beb4405f91a075a564c5c276ab061a6c5e8b0c18d3e3135df265e49e3b.
Hash of previous block is 3294043edb16abe51417fd1ba2d3ecdc497d11c897fdcbc96d6edc8ada661c26.
Data for this block is:
Sender: Sender10
Receiver: Receiver10
Amount: 10000
Block number 10 is added to the blockchain.
Its hash is dc512044c05d96a74fe97b27c374f1eefe0aa7e70dceaacedc691e35eee212f3.
Hash of previous block is eb21c2beb4405f91a075a564c5c276ab061a6c5e8b0c18d3e3135df265e49e3b.
Now, if any of the blocks are modified, the hash for that block will change, and it will not be consistent with the value of the previous hash in the next block. Hence the chain will be broken!
Visit QuantInsti for additional insight on this topic: https://blog.quantinsti.com/blockchain/
Disclosure: Interactive Brokers
Information posted on IBKR Campus that is provided by third-parties does NOT constitute a recommendation that you should contract for the services of that third party. Third-party participants who contribute to IBKR Campus are independent of Interactive Brokers and Interactive Brokers does not make any representations or warranties concerning the services offered, their past or future performance, or the accuracy of the information provided by the third party. Past performance is no guarantee of future results.
This material is from QuantInsti and is being posted with its permission. The views expressed in this material are solely those of the author and/or QuantInsti and Interactive Brokers is not endorsing or recommending any investment or trading discussed in the material. This material is not and should not be construed as an offer to buy or sell any security. It should not be construed as research or investment advice or a recommendation to buy, sell or hold any security or commodity. This material does not and is not intended to take into account the particular financial conditions, investment objectives or requirements of individual customers. Before acting on this material, you should consider whether it is suitable for your particular circumstances and, as necessary, seek professional advice.
Disclosure: Bitcoin Futures
TRADING IN BITCOIN FUTURES IS ESPECIALLY RISKY AND IS ONLY FOR CLIENTS WITH A HIGH RISK TOLERANCE AND THE FINANCIAL ABILITY TO SUSTAIN LOSSES. More information about the risk of trading Bitcoin products can be found on the IBKR website. If you're new to bitcoin, or futures in general, see Introduction to Bitcoin Futures.